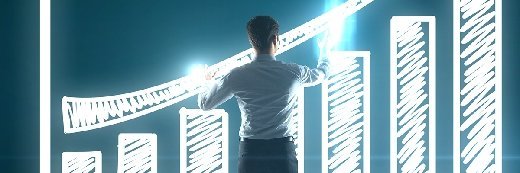
peshkova - Fotolia
Using PowerShell to create XML documents
Administrators can avoid introducing errors when building an XML file by using PowerShell to construct the document.
Traditionally, the exercise to create XML documents by hand has been a pain. The specific, required structure of an XML document means creating one manually can lead to errors. Mistype a character or two, forget to close a bracket or don't declare the namespace correctly and the entire document won't verify. It's always best to rely on code to create an XML document. Code doesn't have clumsy fingers to make typos and doesn't forget to add specifics tags.
In PowerShell, there are a few ways to create XML documents. One of the best methods is to use the XMLTextWriter class. Other techniques exist to convert existing text files into XML documents, but that text file has to exist first. Using the XMLTextWriter class, this isn't necessary.
This tip will explain how to create a well-formed XML document. At the end, the XML document should look like this:
<?xml version="1.0"?>
<!—Car list-->
<Cars Owner="Jay Leno">
<Car VIN="123567891">
<Make>Ford</Make>
<Model>Taurus</Model>
<Year>2012</Year>
</Car>
<Car VIN="123555567891">
<Make>BMW</Make>
<Model>328i</Model>
<Year>2015</Year>
</Car>
</Machines>
First, instantiate the XmlTextWriter object using the New-Object cmdlet. Since the XmlTextWriter object needs to know where to create the XML file, we have to pass the XML file path to its constructor:
$xmlWriter = New-Object System.XMl.XmlTextWriter('C:\Cars.xml',$Null)
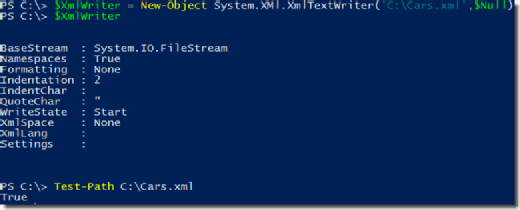
As seen in Figure 1, the moment the object is created, it immediately creates the XML file. At this point, it is a blank text file.
For an XML file that is easier to read, we should apply formatting rules. Without this formatting, all the nodes will be compacted and hard to read. While PowerShell can read the nodes, it's not easy for us humans. Because of this, I like to indent all my nodes with tabs.
$xmlWriter.Formatting = 'Indented'
$xmlWriter.Indentation = 1
$XmlWriter.IndentChar = "`t"
Next, create a well-structured XML file by writing the declaration with version 1.0.
$xmlWriter.WriteStartDocument()
Next, make a comment about the Cars tag.
$xmlWriter.WriteComment('Car List')
Then create the root element called Cars.
$xmlWriter.WriteStartElement('Cars')
Next, set XML attributes using the WriteAttributeString() method. Then create an Owner attribute to signify who owns all of these cars.
$XmlWriter.WriteAttributeString('Owner', 'Jay Leno')
When the root element is created, add a couple elements (cars) inside by using WriteStartElement(). This tells PowerShell that everything we now add will go into a new element.
$xmlWriter.WriteStartElement('Car')
Next, add the VIN attribute to the first Car element and then create a few nodes inside of the Car element to represent various attributes.
$xmlWriter.WriteAttributeString('VIN', '123567891')
$xmlWriter.WriteElementString('Make','Ford')
$xmlWriter.WriteElementString('Model','Taurus')
$xmlWriter.WriteElementString('Year','2012')
After adding information about this particular Car element, end this element by using the WriteEndElement() method.
$xmlWriter.WriteEndElement()
Now, let's just add another Car element.
$xmlWriter.WriteStartElement('Car')
$xmlWriter.WriteAttributeString('VIN', '123555567891')
$xmlWriter.WriteElementString('Make','BWM')
$xmlWriter.WriteElementString('Model','328i')
$xmlWriter.WriteElementString('Year','2015')
Next, close the root element Cars.
$xmlWriter.WriteEndElement()
And finally, close the document, flush whatever is in the internal buffer and close the stream to the file releasing control.
$xmlWriter.WriteEndDocument()
$xmlWriter.Flush()
$xmlWriter.Close()
You should now have a well-formed XML document. To verify, let's read the XML file and to see if everything looks OK.
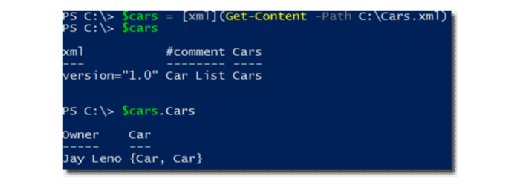
As shown in Figure 2, the version, comment, attribute Owner and two Car nodes have been added. Success!
Using XmlTextWriter is the best way to create XML documents. It has the functionality to perform whatever you need to do to the XML document without having to worry about ensuring everything is perfectly formatted with a plain-text editor.